UVa 210 Concurrency Simulator 【Deque】
题目大意:
本题需要你模拟一些简单程序,每一个程序有以下5种指令:
- var = val,给变量赋值,简单起见保证变量名为一个字母,变量为所有进程共用,并且初始为0,保证val是不大于100的正整数;
- print var,输出变量var;
- lock对所有变量申请独占访问(不影响赋值和打印)
- unlock解除独占访问
- end结束程序
以上指令分别消耗t1,t2,t3,t4,t5的时间,一开始进程按照输入顺序依次插入到等待队列中,每次从等待队列队首选择一个进程执行。每个进程有一个配额(限定时间)Q,当配额用完时,该进程会在执行完当前语句后被立即插入到一个等待队列尾部中。
但是lock语句和unlock语句会改变进程的执行顺序。当一个程序执行了lock语句,其他进程再执行到lock语句时会被立即插入到一个阻止队列队尾,当程序执行到unlock语句时,阻止队列的队首的第一个进程会被立即插入到等待队列队首。
解题思路:
总体看来需要两个队列分别存取lock的进程和ready(等待)的进程。存取lock进程的队列只需要插入到队尾和从队首取这两种操作即可,普通队列就可完成;存取ready进程的队列除从队首取元素外,既需要插入到队尾又需要插入到队首,故需要双端队列来实现。然后模拟整个过程即可。
具体实现时先把所有操作都存下来,并记录每个起始进程的坐标,然后模拟进程的运行,执行相应语句就行了。
Mycode:
1 |
|
UVa 514 Rails【stack】
题目大意:
编号为1 ~ n 的n个元素依次入栈,问给出的出栈顺序是否是合法的。
解题思路:
直接模拟比对即可。
Mycode:
1 |
|
UVa 442 Matrix Chain Multiplication【stack】
题目大意:
给出$t$个$n \times m$的矩阵,问给出的矩阵乘法表达式是否合法,合法的话进行了多少次普通乘法。
解题思路:
$p \times q$ 的矩阵 只能与$q \times r$的矩阵相乘,此时进行的乘法次数为$p \times q \times r$次,再与其他相乘的时候就是次数之和了。
表达式用栈来模拟就好了。
Mycode:
1 |
|
UVa 11988 Broken Keyboard (a.k.a. Beiju Text) 【链表】
题目大意:
Albert的键盘出现了奇妙的故障,所有键都会正常的工作,但是键盘上的Home以及End键有时候会莫名其妙的自己按下。但是盲打很熟练的他一般习惯关闭显示器打字,因为这样很酷。
现在他正在打一段文本,假设你已经知道这段文本以及Home和End键会什么时候出现故障自行按下。请你编写一个程序,求出他最后打出的文本。
解题思路:
- 用数组保存这段文本,然后设置一个变量pos保存光标位置,这样输入一个字符相当于在数组中插入一个字符,这时把后面的字符向后移动就可以了。 但是这样会超时。
- 采用链表,每输入一个字符就把它存起来,设输入的字符串是s[1~n],则可以用next[i]表示在当前显示屏中s[i]右边的字符编号(在s中的下标)。 具体做法:用变量记录当前位置和最后一个字母的位置,模拟移动、插入过程。方便起见,开头设置一个虚拟结点。
链表:
用数组模拟链表的方法:一个数组记录原本的值,另一个数组记录该位置的下一个位置的下标。
Mycode:
1 |
|
UVa 12657 Boxes in a Line【链表】
题目大意:
现有从左到右编号为1 ~ n的n个盒子摆成一行,分别执行如下4种指令的某些指令,问执行后所有奇数位置的盒子的编号之和。
指令有:
- 1 x y 将盒子x移动到盒子y的左边 (如果x已经在y的左边,忽略此指令)
- 2 x y 将盒子x移动到盒子y的右边 (如果x已经在y的右边,忽略此指令)
- 3 x y 交换盒子x和y的位置
- 4 反转整条链
解题思路:
采用双向链表模拟整个过程。
解后有感:
小技巧: 对于过程4我们可以不用模拟反转的过程,用个标志进行记录是否进行了反转就可以。如果反转了,那1、2操作将变为相应的2、1操作,3操作无影响,最后结果记录偶数的位置就行。
关于代码:感觉自己写的十分“朴素”,写完后虽然能AC,但日后再看起肯定不好理解,而且调试时也不那么得心应手。看完LRJ大爷的代码,真真感觉到了自己菜爆。
- 首先链表的连接操作用一个函数表示,减少代码量的同时增加了美观性,方便调试。
- 链表建立时建的循环链表,而且一个循环left和right都构建完了,还是比我的好看多了。
- 特判交换位置时的那部分,将两种情况合为一种(其实就是xy的位置不同,完全能想到的),也就减少了代码量。
- 最后的计算只计算正着看的奇数位的和,反转时直接用总和减去此时的值。
日后注意的地方:
- 多次用到一种计算时写成函数
- 先思考后写代码,逻辑弄清后再写
- 能用1行代码写清楚的地方就不用2行。
最后附上我和LRJ大爷的代码 对比感受一下。
Mycode:
1 |
|
LRJ’s code:
1 | // UVa12657 Boxes in a Line |
UVa 679 Dropping Balls 【二叉树编号】
题目大意:
一棵二叉树最大深度为D,所有叶子的深度都相同,所有结点从上到下从左到右编号为1,2,3,……,$2^D - 1$。在结点1处放个小球,它会往下落。每个结点处都有一个开关,初始时全部关闭,当有小球落到一个开关时其状态就会改变。当小球到达结点时若此开关开着则往左走,否则往右走,直到走到叶子结点。
一些球从1处依次往下落,问第i个会落在哪。
解题思路:
很明显对于一个结点k而言,其左子结点为$2 \times k$,右子结点为$2 \times k + 1$。因为每个球最终都会落在叶子结点上,所以前两个一定是第一个落在左子树上,第二个落在右子树上。推广到一般来看,只需知道小球是第几个落在根的子树里的就能知道它下一步是往左还是往右了。
具体的,拿第一步为例,如果一开始i & 1
,那它就往左,此时位置变为1 << 1
,在新的“根结点”处它就成了第i + 1 << 1
个来到此结点的小球了;同理,如果一开始!(i & 1)
,那它就往右,位置变为1 << 1 | 1
,新的“根结点”处变成第i << 1
个到此位置的小球。然后我们就可以反复执行此过程直到小球落到叶子结点了。
Mycode:
1 |
|
UVa 122 Trees on the level 【二叉树的层次遍历】
题目大意:
输入一颗二叉树,请你从上到下、从左到右的顺序输出各个结点的值。输入的格式详情参照题目说明。
解题思路:
我们可以将树上结点编号,然后把二叉树存到数组里。结点最多256个,如果他们形成一条链的话,最后一个结点的编号高达$2^{256} - 1$,数组根本开不下。- 采用动态结构,将需要的建立新的结点,然后将其组织成一棵树。
PS:下面的代码是参照LRJ大爷给出的代码写的,随着学习的深入越加觉得LRJ大爷真是tql!
Mycode:
1 |
|
UVa 548 Tree 【二叉树的递归遍历】
题目大意:
给出一颗点带权的二叉树的中序和后序遍历,找一个叶子使得它到根的路径上的权和最小。如果有多解,该叶子本身权应尽量小。
解题思路:
后序遍历的最后一个字符是根,我们在中序遍历中找到它,然后就可以找到左右子树的中序和后序遍历了。根据这个把树通过递归构建出来,然后再执行一次递归遍历来找最优解就OK了。
Mycode:(参考紫书)
1 |
|
UVa 839 Not so Mobile【二叉树的递归遍历】
题目大意:
输入一个树状天平,根据力矩相等原则判断是否平衡。采用递归方式输入,0表示中间结点。
解题思路:
其实可以直接做,就是看怎么写写的既简洁又高效,直接保留书上给的代码了,就不献丑了。。
LRJ’s code:
1 | // 算法:在“建树”时直接读入并判断,并且无须把树保存下来 |
UVa 699 The Falling Leaves【二叉树的递归遍历】
题目大意:
给一颗二叉树,每个节点都有一个水平位置,左子结点在它左边一个单位,右子结点在它右边一个单位。按照先序遍历的方式输入,请你从左到右输出每个水平位置的所有结点的权值之和。
解题思路:
按照递归建树的思想,输入的同时直接进行记录,真正的树不必记录下来。
Mycode:
1 |
|
UVa 297 Quadtrees【四叉树】
题目大意:
给两棵四分树的先序遍历,求二者合并之后(黑色部分合并)黑色像素的个数。p表示中间结点,f表示黑色(full),e表示白色(empty)。
解题思路:
根据前面二叉树递归建树的思想建四叉树,然后统计就可以了。
然后,这个题有毒吧?我写的为啥过不了啊??uDebug上的数据AC的代码过不了,我的能过,这都什么啊,找了一上午的问题,愣是没找出来。难受啊。把输入的字符串定义为全局变量直接访问而非通过参数传递访问就AC了。
UPD:经过一下午的对比,发现因为我字符数组定义的位置不同,返回的结果不一样?定义在最上面AC,中间RE,最下面WA,真是神了。。
Mycode(Wrong Answer):
1 |
|
Mycode(Accepted):
1 |
|
LRJ’s code:
1 | // UVa297 Quadtrees |
UVa 572 Oil Deposits 【DFS】
题目大意:
求给定的图中有多少个@的连通块,每个@与它周围相邻的八个小块是联通的。
解题思路:
DFS入门题。
可以用vis标记访问过的内容,也可以直接将访问过的改为无关的符号(用过一次不再用时可以这样做)。
Mycode:
1 |
|
UVa 1103 Ancient Messages 【DFS】
题目大意:
编程识别6种古代象形文字,每组数据包含一个H行W列的字符矩阵,每个字符矩阵为4个相邻像素点的十六进制(eg: $10011100$ 对应字符就是$9C$)。转化为2进制后,1代表黑点,0代表白点。
输入满足:
1.不会出现上述6种符号外的其他符号。
2.输入至少包含一个符号,且每个黑像素都属于一个符号。
3.图像中的每个黑色像素都是有效象形文字的一部分。
4.象形文字不接触也不包含。
5.如果两个黑像素有公共顶点那么他们一定有公共边。
6.所有符号形状和给出的图形拓扑等价(可以随意拉伸但不能拉断)。
解题思路:
1.16进制转换为2进制,建图。
2.观察发现每个图都有一个与其他图像完全不同的特征——洞的个数,可以把此作为为突破点。
3.第一遍把所有象形文字“抠”出来,具体操作是将所有为0的部分标记一下。
4.第二遍开始给每个象形文字的洞标号。具体操作是找没被标记的部分开始DFS,将第i个图的洞全部标记为i号。
5.第三遍数洞的个数。因为上一步已经将第i号的洞标记为了i,这时就遍历整个图看有几个连通块编号为i即可。
(自己的代码不够精简,很明显的DFS0和DFS1是可以合并的,加个if判断就好了)
【小技巧】因为步骤一中第一个非象形文字的洞的0不好确定,所以我们建图时可以从(1,1)开始存储给出的图,这样(0,0)必定是非象形文字的洞的0了。
Mycode:
1 |
|
UVa 816 Abbott’s Revenge 【BFS】
题目大意:
在一个最多包含9 * 9个交叉点的迷宫中,输入起点、离开起点时的朝向和终点,求一条最短路(多解时输出任意一条)。
解题思路:
这个相对于一般的最短路问题而言多了方向,因此无法用最短路的算法求解,这时考虑到使用BFS来解决。
对于路径的打印,可以采用递归的方式打印。如果最短路很长的话递归可能会引起栈的溢出,此时改用循环,用vector记录路径。
PS:一开始接触这个题目时毫无头绪,也是参照了书上的内容一点一点扣了很久才弄明白的。
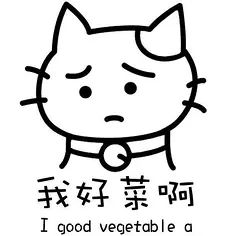
Mycode:
1 |
|
UVa 10305 Ordering Tasks 【拓扑排序】
题目大意:
给出n个任务和m个限制条件,要求任务i必须在任务j之前完成。请给出一个合适的任务完成顺序。
解题思路:
拓扑排序裸题。
Mycode:
1 |
|
UVa 10129 Play on Words 【欧拉回路】【并查集】
题目大意:
给出n个单词,能否可以把他们排成一列,使得每个单词的第一个字母和上一个单词的最后一个字母相同。
解题思路:
将字母看做结点,单词看成有向边,那么当且仅当图中有欧拉回路的时候问题有解。
有向图存在欧拉回路的条件有两个:1. 底图(即忽略方向后得到的无向图)联通 2.度数满足欧拉道路的条件。
这里判断条件1时用的并查集,判断条件二时直接记录的度数。
欧拉回路
- 无向图中从一个结点出发走出一条道路,每条边恰好经过一次,这样的道路称为欧拉道路。
- 度:无向图里,度为一个顶点关联边的个数。
- 如果一个无向图是联通的,且最多有两个度数为奇数的点,那么一定存在欧拉道路。如果有两个奇点,则必须从一个奇点出发到另一个奇点终止,其余情况,则可以从任意点出发,最终一定会回到该点(称为欧拉回路)。
–> 类推出有向图:
- 最多只能有两个点的入度不等于出度,而且必须是其中一个点的出度恰好比入度大1(起点),另一个的入度比出度大1(终点)。
- 前提条件:忽略方向后,图是联通的。
Mycode:
1 |
|
UVa 10562 Undraw the Trees 【树的结构】
题目大意:
给出一个多叉树,将其转化为括号表示法。
解题思路:
直接在二维字符数组中递归,无需建树。
MyCode:
1 |
|